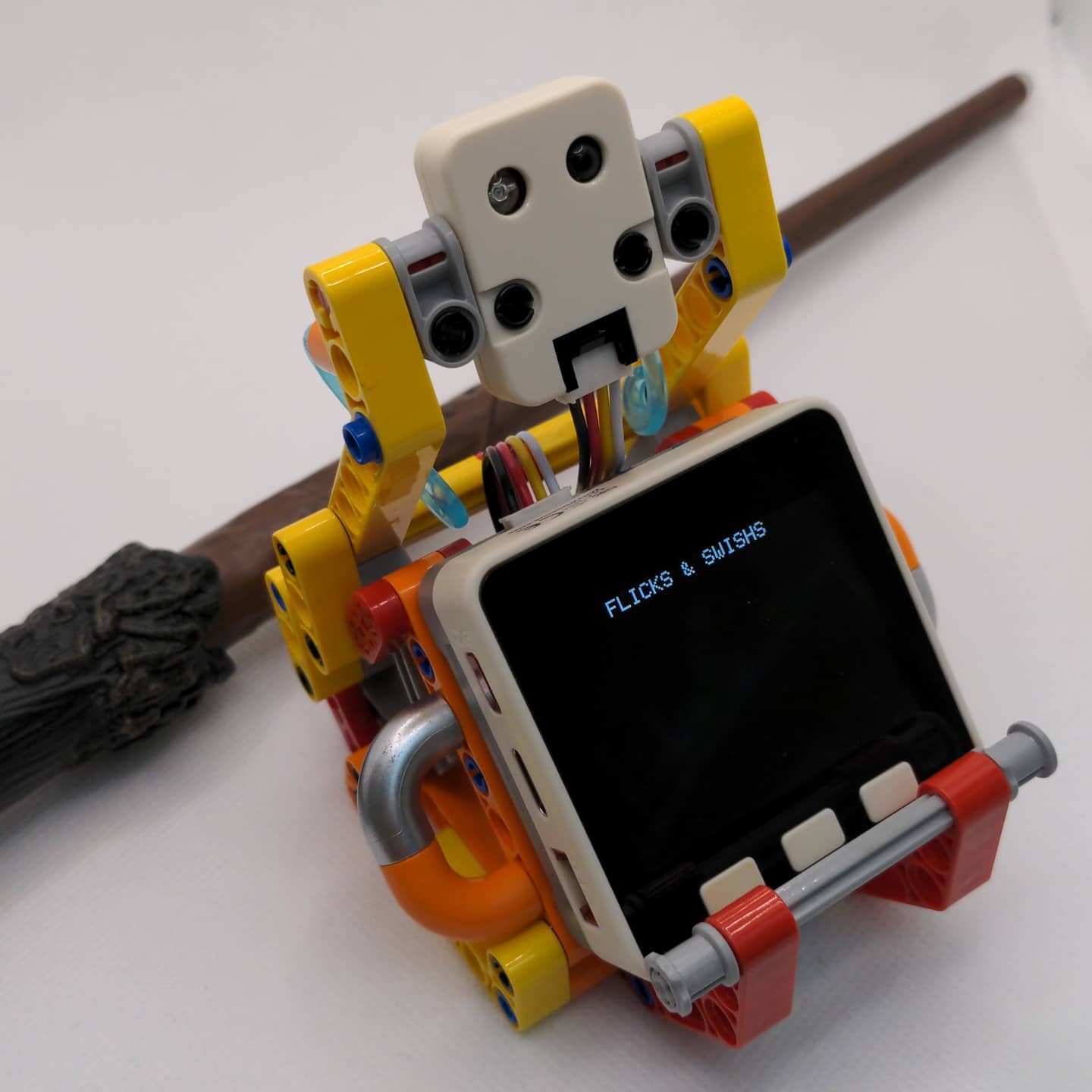
I had done a few projects in the past using IR remote as a way of wireless communication. I used FLIRC USB (https://flirc.tv/more/flirc-usb). It is essentially a USB IR receiver that plugs into your computer and turns IR signals into specific keystrokes. The great thing about FLIRC is that once you configured it on a computer with its application, you can use it anywhere with a USB port. The only downside is that the FLIRC USB only offers six customizable inputs (up, down, left, right, enter, and back) because it is meant to be used for media playback. I just figured out a way to do it with M5Stack and the IR Unit now.
IRremoteESP8266 Library also works for ESP32 (M5Stack)
https://github.com/crankyoldgit/IRremoteESP8266
I am using the M5Stack Core + IR Unit (Port B), so have to change the pin to 36.
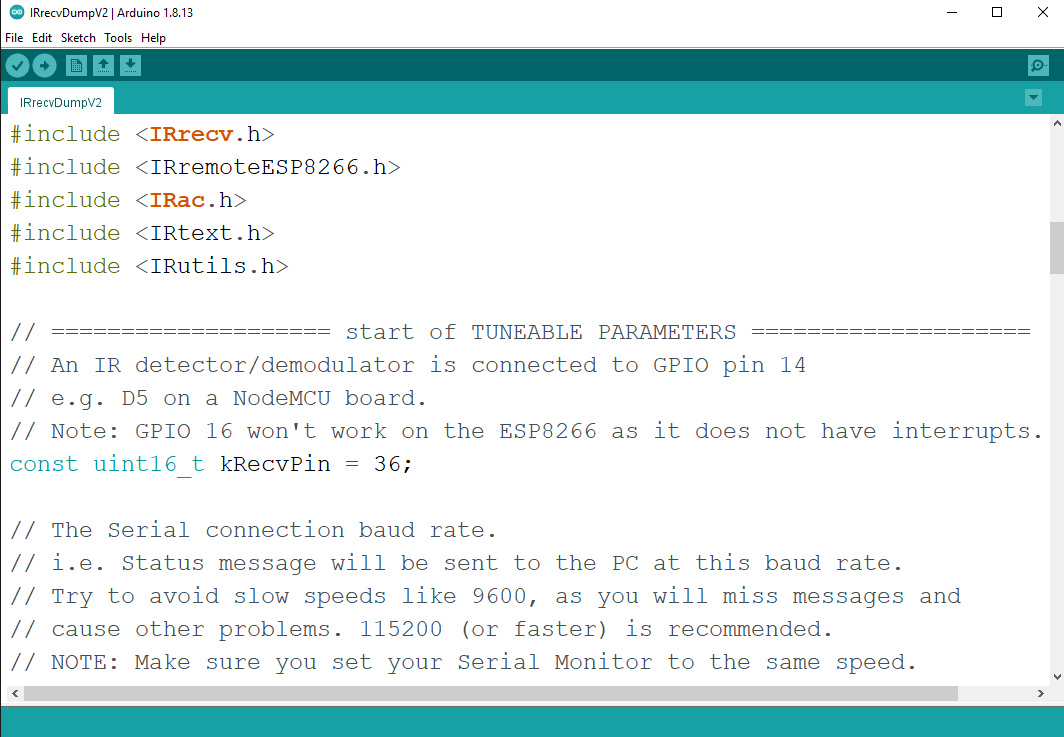
Upload it to M5Stack, get the readout (Code) from the serial monitor. Since I don’t need to recreate the IR signal, the Code here will work just fine.
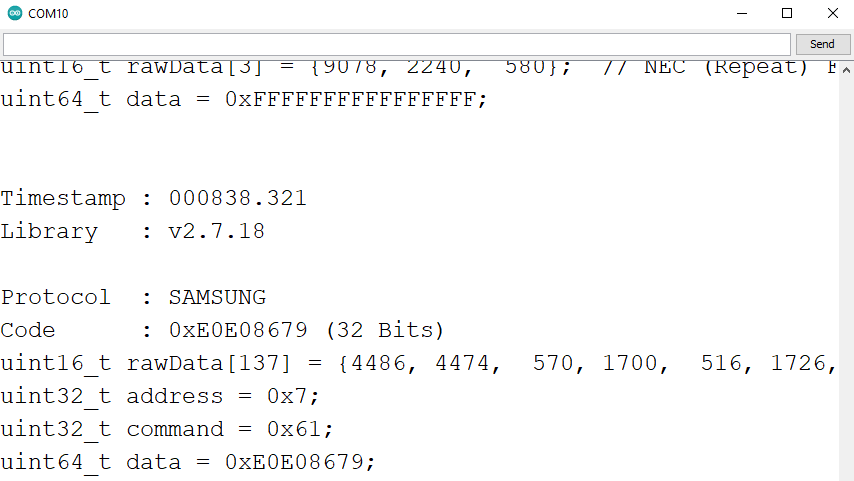
Now, M5Stack can read the remote and react!
In order to work with result.value, I need a uint64_t to String helper method to make the comparison easier for me.
String u64ToS(uint64_t input) { String result = ""; uint8_t base = 10; do { char c = input % base; input /= base; if (c < 10) c +='0'; else c += 'A' - 10; result = c + result; } while (input); return result; }
or
String print64(uint64_t n) { char buf[21]; char *str = &buf[sizeof(buf) - 1]; String sdata = ""; *str = '\0'; do { uint64_t m = n; n /= 10; *--str = m - 10 * n + '0'; } while (n); sdata += str; return sdata; }